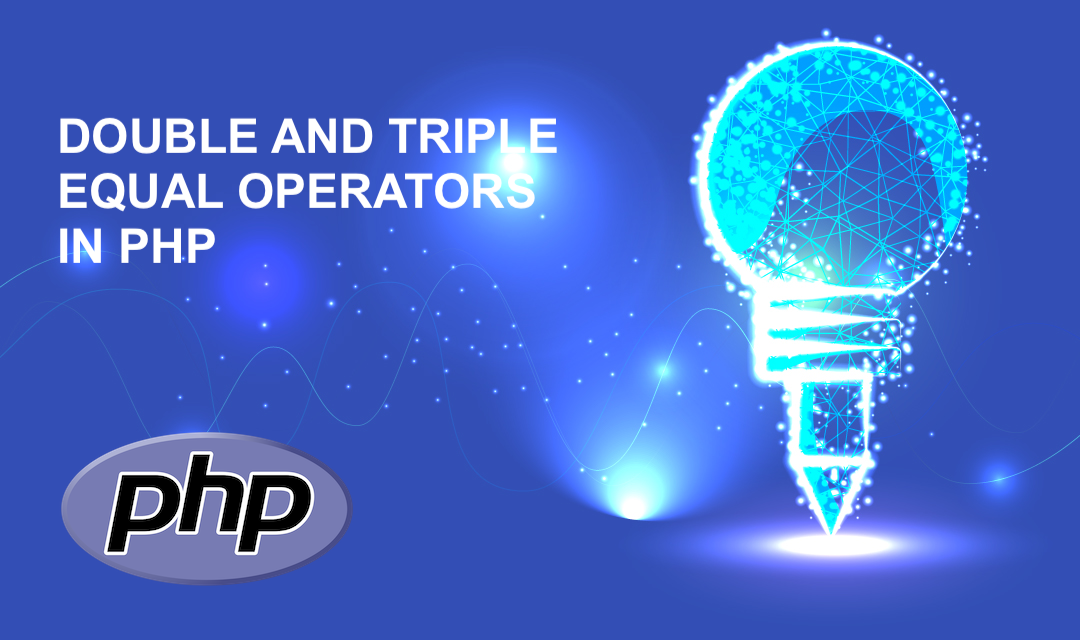
Even if you're a seasoned programmer, you may not have seen triple equals operator in most programming languages. In PHP, the triple equals (===) operator was introduced in version 4 and it checks for equality similar to the double equals (==) operator but also checks the type of variable. Here are the differences between single, double, and triple equals operators.
Equal (=) Assignment Operator
A single equals operator (=) is an assignment operator - take what's on the right as an expression and save it in the variable named on the left.
$a = 5; // A is assigned an integer value 5.
Comparison Operators
1. == (Equality Operator)
In PHP, == and === are comparison operators, but they have different behaviors:
A double equals operator (==) is a comparison operator and it tests the value (variable, expression, or constant) of left to the right for equality. If the values are the same, it returns true.
$a = 5; $b = "5"; if ($a == $b) echo "same"; // Returns TRUE
2. === (Identity Operator)
A triple equals operator (===) is a comparison operator and it tests the value (variable, expression, or constant) of left to the right for identicalness. The value of left and right have to be equal AND the type has to be equal as well. (i.e. both are strings or both are integers).
$a = 5; $b = "5"; if ($a === $b) echo "same"; // Returns FALSE
Conclusion
- Use == for loose equality checking with type coercion.
It's generally recommended to use === for most comparisons to avoid unexpected type coercion behaviors. However, there might be cases where == is suitable, depending on the specific requirements of your code.
Share this post
Leave a comment
All comments are moderated. Spammy and bot submitted comments are deleted. Please submit the comments that are helpful to others, and we'll approve your comments. A comment that includes outbound link will only be approved if the content is relevant to the topic, and has some value to our readers.
Comments (0)
No comment