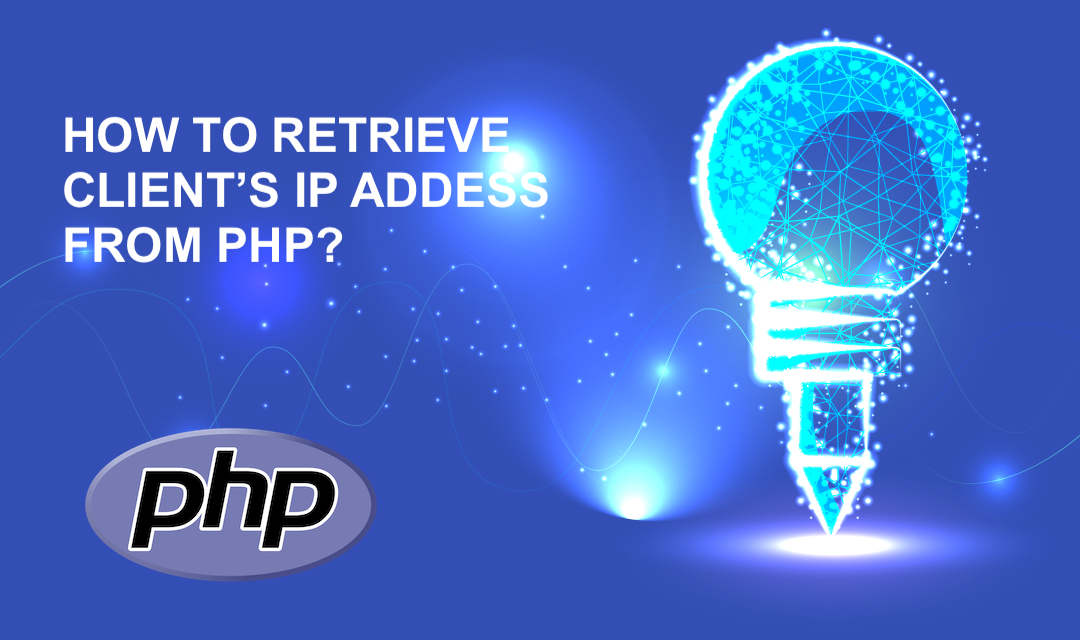
There are a number of array name/value pairs provided in the PHP's "predefined" $_SERVER variable, which you can use to extract client's IP address. Some of the IP-related $_SERVER parameters retrieved from the web server are passed by the client in the form of HTTP header, so they can be easily spoofed. Also, some of the HTTP_X_FORWARD* parameters expected to be filled by proxy servers may not always be there as not all proxy servers follow best practices.
The most reliable source of IP address from the $_SERVER variable is REMOTE_ADDR, and all others mentioned below can be easily spoofed by a remote client. So, if you are dealing with visitors that you cannot trust (i.e. Internet Users), be sure to use $_SERVER['REMOTE_ADDR'] and get the IP address of the remote machine. You may not obtain the true IP address of the clients sitting behind the
If you can trust your clients, you may use the code below to extract IP address of the clients sitting behind a proxy server.
function getRemoteIpAddress(){ $keys = array( 'HTTP_CLIENT_IP', 'HTTP_X_FORWARDED_FOR', 'HTTP_X_FORWARDED', 'HTTP_X_CLUSTER_CLIENT_IP', 'HTTP_FORWARDED_FOR', 'HTTP_FORWARDED', 'REMOTE_ADDR'); foreach ($keys as $key){ if (array_key_exists($key, $_SERVER)){ foreach (explode(',', $_SERVER[$key]) as $ip){ $ip = trim($ip); if (filter_var($ip, FILTER_VALIDATE_IP, FILTER_FLAG_NO_PRIV_RANGE | FILTER_FLAG_NO_RES_RANGE) !== false){ return $ip; } } } } }
The value of HTTP_X_FORWARDED_FOR is set by a Proxy Server and they may contain a comma-separated list of IP addresses if multiple proxies are involved. All HTTP_* parameters passed in the $_SERVER variable can be manipulated by the client machine, so they should not be trusted.
References
Share this post
Leave a comment
All comments are moderated. Spammy and bot submitted comments are deleted. Please submit the comments that are helpful to others, and we'll approve your comments. A comment that includes outbound link will only be approved if the content is relevant to the topic, and has some value to our readers.
Comments (0)
No comment