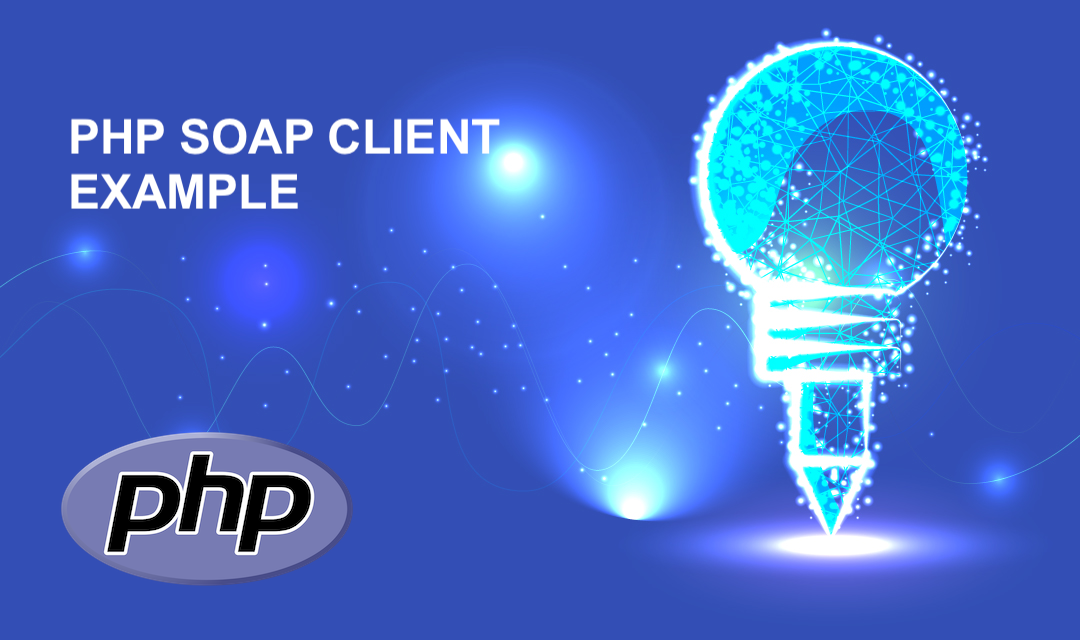
Using the SoapClient in PHP allows you to interact with SOAP (Simple Object Access Protocol) web services. Here's a basic guide on how to use the SoapClient in PHP.
1. What is SOAP?
SOAP is an XML-based communication protocol designed to exchange information between applications. The protocol itself is not tied to a particular operating system or programming language, so the client and server running any platform written in any programming language can exchange soap messages. Web service is gaining popularity, and there are quite a few protocols available but SOAP is probably one of the most popular protocols used today. SOAP server publishes a service over an HTTP, and the SOAP client consumes the service.
2. How do I enable SOAP on my PHP installation?
PHP made a first attempt to implement SOAP protocol via a SOAP extension, and it implements SOAP 1.1 and 1.2 protocols as well as WSDL 1.1 specifications. You'll need libxml and php-soap installed on your platform. On Linux, you may install libxml and php-soap with the yum command and enable the SOAP extension in php.ini. By installing the php-soap, it places the SOAP extension in the PHP modules folder and enables it the next time PHP is loaded.
3. PHP SOAP Extension Functions
You may use PHP SOAP Reference for a complete SOAP resource with installation requirements, and available Client and Server functions. The following are the most notable SoapClient functions used to implement SOAP client.
SoapClient->__construct() - constructs a new SoapClient object SoapClient->__soapCall() - Calls a SOAP function SoapClient->__getFunctions() - Returns list of SOAP functions SoapClient->__getLastRequestHeaders() - Returns last SOAP request headers SoapClient->__getLastRequest() - Returns last SOAP request SoapClient->__getLastResponseHeaders() - Returns last SOAP response headers SoapClient->__getLastResponse() - Returns last SOAP response
4. SOAP Example Code
An example of SOAP service would be an USPS address validation. A SOAP client may request to a SOAP server whether a given postal address is valid, and if not it may request for a recommended correction (if available).
class AddressClient extends SoapClient { private $wsdl = "http://www.webtrafficexchange.com/soap/example?wsdl"; private $location = "http://www.webtrafficexchange.com/soap/addressInterface"; private $connection_timeout = 300; // 5 minutes function __construct() { $options = array('connection_timeout' => 300, 'soap_version' => SOAP_1_2 ); parent::__construct($this->wsdl, $options); } function validateAddress($requestXml) { return $this->__doRequest($requestXml, $this->location, "validateAddress", SOAP_1_2); } }
Share this post
Leave a comment
All comments are moderated. Spammy and bot submitted comments are deleted. Please submit the comments that are helpful to others, and we'll approve your comments. A comment that includes outbound link will only be approved if the content is relevant to the topic, and has some value to our readers.
Comments (0)
No comment