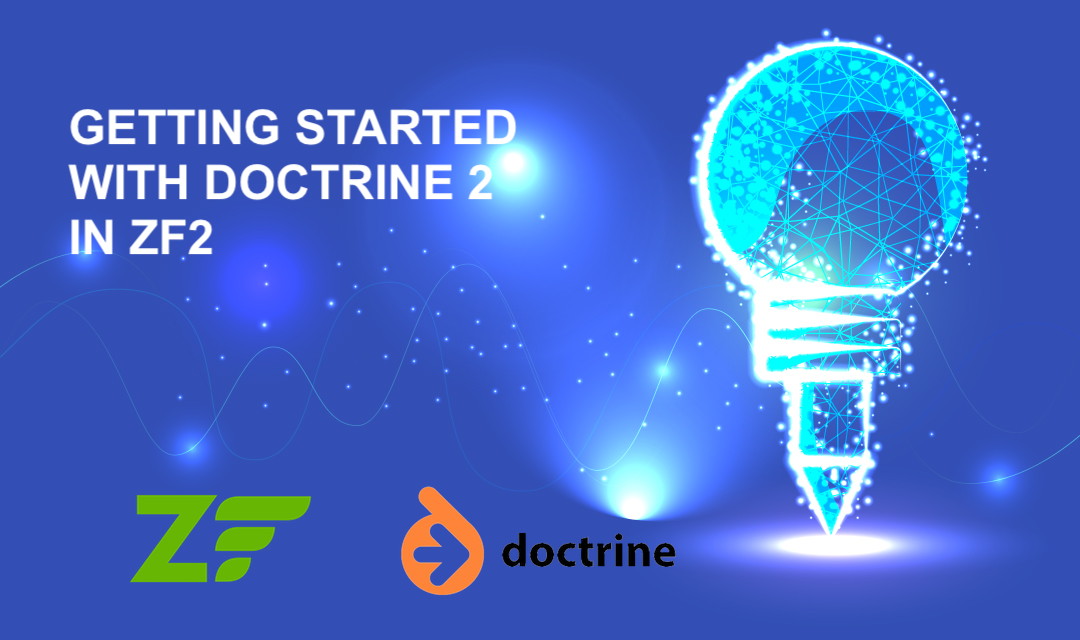
Using Doctrine as an ORM to persist your data significantly lowers your overhead in managing your database. However, if you're just getting started with Doctrine, you'll have to invest in moderate time to overcome the learning curve. Here are some of not so obvious tricks you'll have to learn as a beginner to utilize Doctrine.
Q. How do you install ZF2 doctrine modules?
There are two doctrine modules to install: DoctrineModule and DoctrineORMModule. You cannot just clone the Git projects from GitHUP to install those two modules. Instead, you'll have to use Composer to install the modules.
First you'll have to move to the ZF2 project folder where the "vendor" folder is residing. The DoctrineModule and DoctrineORMModule will be installed in the "vendor" folder, so you must initiate the installation from the ZF2 project folder. The installation will also create the vendor/autoload.php which will resolve all dependencies.
$ cd {ZF2 Project Folder} $ curl -sS https://getcomposer.org/installer | php $ php composer.phar require doctrine/doctrine-module:0.* php composer.phar require doctrine/doctrine-orm-module:0.*
Q. How do you assign DateTime to a Doctrine Object? Use \DateTime object.
$object->myDate = new \DateTime();
Q. How do you retrieve the "last Insert Id" of an object that just persisted?
$em->persist($foo); $em->flush(); $foo->id; // Or, $user->getId(); if you have the getter method defined.
Q. How do you update a record in Doctrine 2?
$user = $em->find('Entity\User', 1); $user>setLastName('Doe'); //Flushing changes to the database (triggers SQL updates) $em->flush(); // You may also invoke the merge method. $em->merge($user);
Q. How do you execute native SELECT SQL statements?
With NativeQuery you can execute native SELECT SQL statements and map the results to Doctrine entities or any other result format supported by Doctrine.
use Doctrine\ORM\Query\ResultSetMapping; $sql = "SELECT userid, name, email FROM users WHERE name='mike'"; $om = $this->getServiceLocator()->get('Doctrine\ORM\EntityManager'); $rsm = new ResultSetMapping(); $rsm->addEntityResult('Application\Entity\User', 'u'); // Entity Alias, Table Column name, Entity Field name $rsm->addFieldResult('u', 'userid', 'id'); $rsm->addFieldResult('u', 'name', 'name'); $rsm->addFieldResult('u', 'email', 'email'); $query = $om->createNativeQuery($sql, $rsm); $users = $query->getResult();
Share this post
Leave a comment
All comments are moderated. Spammy and bot submitted comments are deleted. Please submit the comments that are helpful to others, and we'll approve your comments. A comment that includes outbound link will only be approved if the content is relevant to the topic, and has some value to our readers.
Comments (0)
No comment