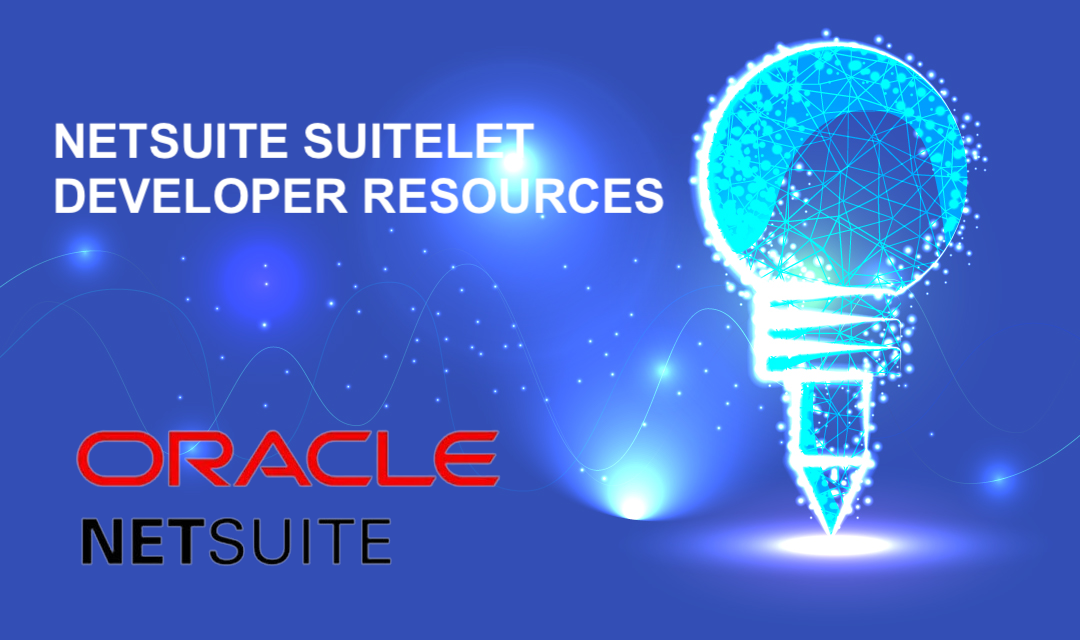
1. Join the SuiteCloud Developer Network To get started with Netsuite development, you'll need a Netsuite account that you can use to communicate with Netsuite. You may sign up with the SuiteCloud Developer Network (SDN) as a Community member, and get a free Netsuite account for exploitation. It may take a day or two to get your account approved.
2. Use NetSuite Script Debugger as much as possible. Write your code in Netsuite Debugger and verify your code work properly before creating a SuiteScript in production.
3. Run Suitelet in the background.
One of the first things we've noticed after implementing Suitelet integration with our legacy application is that it adds roughly 3 additional seconds to process the request. This is troublesome as the resources are customer-facing web pages, and increased load time will significantly lower conversion. To circumvent this type of problem, it is suggested that the Suitelet calls be made in the background. The following code snippet illustrates by example:
Here is a traditional way to call Suitelet with cURL:
$url = "https://forms.netsuite.com"; if ($_SERVER['REQUEST_METHOD'] == "POST") { $ch = curl_init( $url ); curl_setopt($ch, CURLOPT_POST, TRUE); curl_setopt ($ch, CURLOPT_RETURNTRANSFER, TRUE); curl_setopt($ch, CURLOPT_POSTFIELDS, $_POST); // Execute Post $result = curl_exec($ch); // Close connection curl_close($ch); }
*Please note that we need the CURLOPT_RETURNTRANSFER option set to TRUE to grab the "literal" output of the curl_exec() command. If you don't, you may get an additional status code appended to the curl_exec() output.
Instead, you may invoke the Suitelet directly with Linux curl command with background operator, &.
$url = "https://forms.netsuite.com"; $params = ""; foreach ($post as $name => $value) { $params .= "{$name}={$value}&"; } rtrim($params, '&'); exec("/usr/bin/curl -d \"$params\" {$url} >/dev/null 2>&1 &");
*In order to initiate the curl process in the background, you'll need to run the "curl" command in the background with a background Linux operator, "&". See line 8 above.
If you invoke a Suitelet directly with curl as shown in the example above, the error handling must be done in the Suitelet which may be quite troublesome. Also, if you have special characters in the GET parameter values, you'll likely run into issues. To work around this problem, we'll introduce a wrapper PHP file that will invoke the required Suitelet and handle errors gracefully.
$url = "https://www.webtrafficexchange.com/custom.php"; // Custom PHP script $params = ""; foreach ($post as $name => $value) { $value = urlencode($value); $params .= "{$name}={$value}&"; } rtrim($params, '&'); exec("/usr/bin/curl -d \"$params\" {$url} >/dev/null 2>&1 &");
The wrapper PHP file, custom.php, may contain a code similar to:
$url = "https://forms.netsuite.com/app/site/hosting/scriptlet.nl?script=XX&deploy=Y&compid=XYZ&h=SOME_HEX_CODE"; if ($_SERVER['REQUEST_METHOD'] == "POST") { $post = array(); foreach ($_POST as $name => $value) { $post[$name] = urldecode($value); } $ch = curl_init( $url ); curl_setopt($ch, CURLOPT_POST, TRUE); curl_setopt ($ch, CURLOPT_RETURNTRANSFER, TRUE); curl_setopt($ch, CURLOPT_POSTFIELDS, $post); $result = curl_exec($ch); curl_close($ch); }
You'll have to decode the parameter values in the PHP script (line 6) before making a Suitelet call.
4. There are variations of methods available to retrieve a field value from the type of entity you're working with.
nlapiGetFieldValue(); record.getFieldValue(); search.getValue();
The nlapiGetFieldValue() method is available only in Client and User Event SuiteScripts. To retrieve a field value from a NetSuite Record, you'll use getFieldValue() method. When working with search results, you'll use getValue() instead. The three methods above always return value as a string whether it's a date, date/time, or numeric data type. So, when working with those values, you'll have to convert the value to the correct type before manipulating them. There are a number of javascript, and NetSuite functions such as parseInt(), parseFloat(), nlapiStringToDate() available to make this easy.
5. NetSuite is a very comprehensive web application, and sometimes it's difficult to know how to navigate to certain areas of the application. NetSuite Search Box comes to a rescue. Virtually anything can be searched through the search box, and it will guide you to a place where you can make edits and views.
References
Here is a sample chapter on SuiteScript from a book published by John Wiley & Sons. It covers the high-level overview of the SuiteScript with examples. Must see for beginners. If the link below doesn't work for some reason, you may grab one from here.
Writing Your Own Ticket with SuiteScript
More samples of the book from John Wiley & Sons are available on Google Books.
In order to get your hands dirty and feet wet with NetSuite, you'll have to browse NetSuite developer resources and API documentation. Here are a few links to helpful resources.
- SuiteScript Developer and Reference Guide
- NetSuite Developer Resources
- Netsuite PHP Toolkit Documentation
- NetSuite SuiteAnswers ***
- NetSuite User Group
- SuiteTalk Schema Browser (01/2012)
- SuiteCloud (formally known as SuiteFlex) References
- Server SuiteScript Developer's Guide
** Note: I didn't have too much luck with the NetSuite User Group. There isn't that much activity in the NetSuite User Group, but I found SuiteAnswers very useful with examples and tutorials.
Share this post
Leave a comment
All comments are moderated. Spammy and bot submitted comments are deleted. Please submit the comments that are helpful to others, and we'll approve your comments. A comment that includes outbound link will only be approved if the content is relevant to the topic, and has some value to our readers.
Comments (0)
No comment