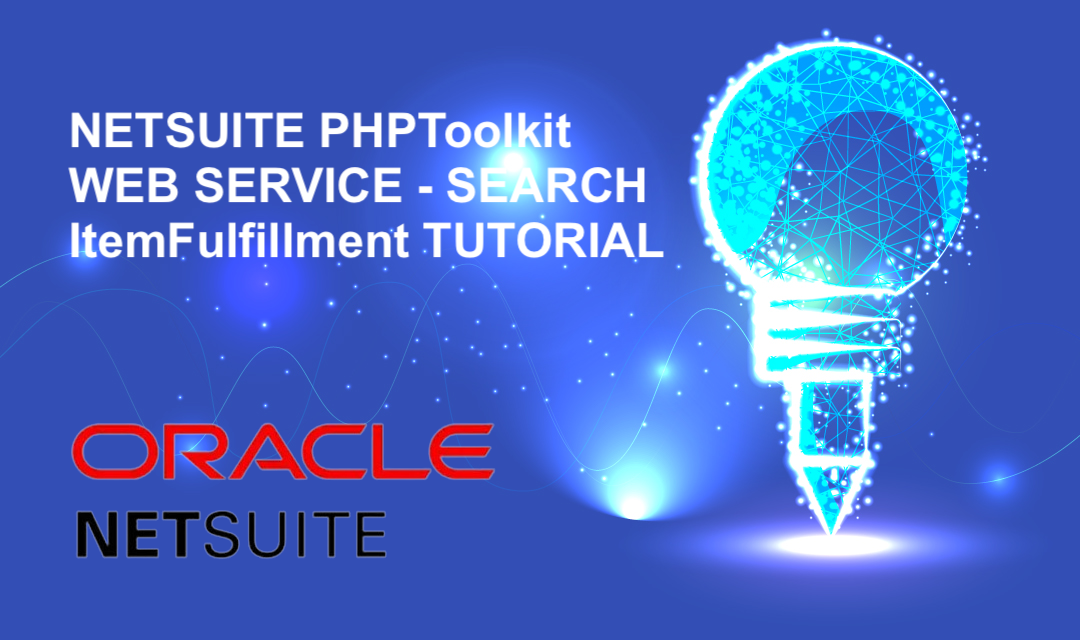
Our business requires that we retrieve Quantum View data from UPS, and create or edit ItemFulfillment records with PackageList and Packages. As described in the earlier articles, we've created a UPS developer access key and set required Quantum View subscriptions for later retrieval of Quantum View events.
I've spent a great amount of time trying to figure out how to retrieve ItemFulfillment record(s) associated with a particular Sales Order. After spending more than a day, I've found a solution after looking through the C# examples. Netsuite documentation on how to use PHPToolKit is somewhat premature, and it requires a lot of digging and trial-and-error to figure out a solution. For those who are new to NetSuite, you may want to check out NetSuite Developer Resources I have compiled for easy reference.
require_once ('PHPtoolkit.php'); require_once ('login_info.php'); class ItemFulfillment { private $so = null; private $data = null; function __construct($soId) { $this->soId = $soId; $this->so = $this->getSalesOrder($soId); } // Retrieve a Sales Order record from NetSuite from the // given Sales Order number. private function getSalesOrder($soId) { global $myNSclient; $id = 0; $myNSclient->setSearchPreferences(false, 10); $params = new nsComplexObject('SearchStringField'); $params->setFields(array('searchValue' => $soId, 'operator' => 'is')); $search = new nsComplexObject("TransactionSearchBasic"); $search->setFields(array ('tranId'=> $params)); $response = $myNSclient->search($search); if ($response->isSuccess) { // We are expecting at most 1 record from this search. $so = $response->recordList[0]; } else { $so = null; } return $so; } public function getSalesOrderId() { if ($this->so === null) { return null; } else { return $this->so->getField('tranId'); } } private function getSalesOrderInternalId() { if ($this->so === null) { return null; } else { return $this->so->getField('internalId'); } } /** * Retrieve the ItemFulfillment record(s) attached to a Sales Order. * */ public function getFromSalesOrder() { global $myNSclient; $id = $this->getSalesOrderInternalId(); $myNSclient->setSearchPreferences(false, 10); $search = new nsComplexObject("TransactionSearchBasic"); $type = new nsComplexObject('SearchEnumMultiSelectField'); $type->setFields(array('searchValue' => array('_itemFulfillment'), 'operator' => 'anyOf')); $so = new nsRecordRef(array('type' => 'salesOrder', 'internalId' => $id)); $createdFrom = new nsComplexObject('SearchMultiSelectField'); $createdFrom->setFields(array('searchValue' => $so, 'operator' => 'anyOf')); $search->setFields(array ('type' => $type, 'createdFrom' => $createdFrom)); $response = $myNSclient->search($search); //print_r($response); return $response->recordList; } /** * Retrieve a ItemFulfillment record from an Internal ID. * * @param String $id -- Internal ID of the ItemFulfillment record */ public function get($id) { global $myNSclient; $recordRef = new nsRecordRef(array('internalId' => $id, 'type' => 'itemFulfillment')); $response = $myNSclient->get($recordRef); return $response->record; } /** * Return packages. * * @param integer $id - ItemFulfillment Internal ID * returns an array of nsComplexObject('ItemFulfillmentPackage'). */ public function getPackages($id) { global $myNSclient; $itemFulfillment = $this->get($id); $packages = null; if ($itemFulfillment != null) { $packageList = $itemFulfillment->getField('packageList'); if ($packageList != null) { $packages = $packageList->getField('package'); } } return $packages; } /** * Add an ItemFulfillment record with Package Info into NetSuite. */ public function add() { global $myNSclient; $id = $this->getSalesOrderInternalId(); echo "Internal ID: " . $id . "\n"; // Package Info $packageFields = array ( 'packageWeight' => 1.0, 'packageTrackingNumber' => '1Z00111122223333' ); $package = new nsComplexObject('ItemFulfillmentPackage'); $package->setFields($packageFields); $packageListFields = array ('package' => $package ); $packageList = new nsComplexObject('ItemFulfillmentPackageList'); $packageList->setFields($packageListFields); // ItemFulfillment Record $itemFulfillment = new nsComplexObject('ItemFulfillment'); $fulfillmentFields = array ( 'createdFrom' => new nsRecordRef ( array('internalId'=>$id, 'type' =>'salesOrder' )), 'packageList' => $packageList ); $itemFulfillment->setFields($fulfillmentFields); $myNSclient->setPreferences(true, false, false, false, true, true); $response = $myNSclient->add($itemFulfillment); if ($response->isSuccess) return true; else{ return false; } } }
For those who may be interested in looking at C# examples, here is the code snippets offered as help.
Retrieving all ItemFulfillment records from NetSuite.
TransactionSearch transactions = new TransactionSearch(); TransactionSearchBasic transactionsBasic = new TransactionSearchBasic(); // Set transaction type SearchEnumMultiSelectField searchItemFullfillmentField = new SearchEnumMultiSelectField(); searchItemFullfillmentField.@operator = SearchEnumMultiSelectFieldOperator.anyOf; searchItemFullfillmentField.operatorSpecified = true; searchItemFullfillmentField.searchValue = new String[] { "_itemFulfillment" }; transactionsBasic.type = searchItemFullfillmentField; transactions.basic = transactionsBasic; SearchResult searchResult = _service.search(transactions);
Searching for a particular ItemFulfillment record associated with a Sales Order.
RecordRef so = new RecordRef(); so.internalId = "Your_SO_Id"; so.type = RecordType.salesOrder; so.typeSpecified = true; SearchMultiSelectField createdFrom = new SearchMultiSelectField(); createdFrom.@operator = SearchMultiSelectFieldOperator.anyOf; createdFrom.operatorSpecified = true; createdFrom.searchValue = new RecordRef[] {so}; transactionsBasic.createdFrom = createdFrom;
Share this post
Leave a comment
All comments are moderated. Spammy and bot submitted comments are deleted. Please submit the comments that are helpful to others, and we'll approve your comments. A comment that includes outbound link will only be approved if the content is relevant to the topic, and has some value to our readers.
Comments (0)
No comment