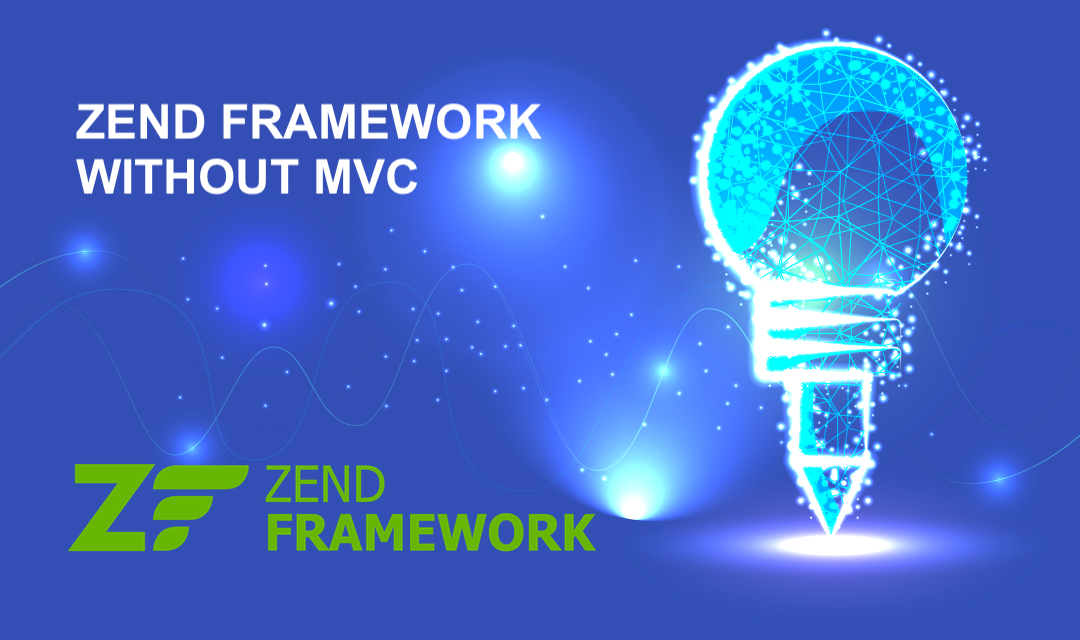
The reasoning for using a framework such as Zend Framework (ZF for short) is to speed up the development process, make the application extensible, and make use of the design patterns such as MVC. I think MVC is great, it separates the models, views, and controllers and makes the entire development process very clean. If MVC is great, why would you use Zend Framework without MVC?
Zend Framework is a loosely coupled (or "glued") component library, which you may use independently without using the entire framework. For example, if you're trying to put up a quick A/B split testing "landing" pages for a website built with Zen Cart, which you'll use with Google Optimizer. Trying to set up a new ZF project on top of an existing website that does not utilize ZF may be an overkill if you're just trying to put up a couple of web pages. Luckily, ZF can be used as a component library to build a couple of web pages without using the entire framework. In this article, I will share my experience in building a webpage containing HTML form and posting it to itself using Zend Components.
Email Subscription Example
I am going to use a very simple example, perhaps too simple to even bother with a framework like ZF. This is just for an illustration purpose, so let's not debate whether it makes sense or not, but focus on how we can make use of the Zend component library to build a self-posting webpage. The webpage will have an HTML form with three input elements, namely "First Name", "Last Name" and "Email Address".
// Include library for ZendFramework set_include_path(implode(PATH_SEPARATOR, array( '/home/sample/library', get_include_path() ))); require_once 'Zend/Config.php'; require_once 'Zend/Form.php'; require_once 'Zend/Mail.php'; require_once 'Zend/View.php'; $$db_config = array( 'database' => array( 'adapter' => 'pdo_mysql', 'params' => array( 'host' => 'db.example.com', 'username' => 'dbuser', 'password' => 'secret', 'dbname' => 'mydatabase' ) ) ); $config = new Zend_Config($db_config); $form = new Zend_Form(); $form->addElement('text', 'fname', array('label' -> 'First Name'); $form->addElement('text', 'lname', array('label' -> 'Last Name'); $form->addElement('text', 'email', array('label' -> 'Email Address', 'required' => true, 'filters' => array('StringToLower'), 'validators' => array( array('NotEmpty', true, array('message' => 'Enter your email address')), array('EmailAddress', true))) ); $form->setView(new Zend_View()); if ($_SERVER['REQUEST_METHOD'] == "POST") { $db = Zend_Db::factory($config->database); ... logic to save posted data to a database. } else { echo $form; }
Conclusion
The Zend Framework (now known as Laminas) is a powerful PHP framework that originally included a full-featured Model-View-Controller (MVC) architecture. However, it is possible to use Zend Framework components without strictly adhering to the MVC structure.
The Zend Framework has been rebranded as Laminas in April 2019. Laminas is now a collection of individual components that can be used independently. When considering the use of Zend Framework components without MVC, it's crucial to understand the specific needs of your project and choose the components that align with those requirements.
Share this post
Leave a comment
All comments are moderated. Spammy and bot submitted comments are deleted. Please submit the comments that are helpful to others, and we'll approve your comments. A comment that includes outbound link will only be approved if the content is relevant to the topic, and has some value to our readers.
Comments (0)
No comment